Assemblies
The assembly is the building block of a VB.NET application. Basically, an assembly is a collection of the types and resources that are built together to provide functionality to an application.
· Assemblies look like dynamic link libraries (.dll) or executable programs (.exe).
· Differ from .exe and .dll files in that they contain the information found in a type library plus the information about everything else needed to use an application or component.
· Includes a mix of Microsoft Intermediate Language (IL) and machine code.
· Invokes the CLR by machine code found in the first several bytes of an assembly file.
· Contains one or more files.
· An application can be composed of one or more assemblies.
· An assembly can be a single portable executable (PE) file (like an .exe or .dll) or multiple PE files and other external resource files such as bitmap files.
Assemblies store metadata (data about the application) and include:
§ Information for each public class or type used in the assembly – information includes class or type names, the classes from which an individual class is derived, etc.
§ Information on all public methods in each class – includes the method name and any return values.
§ Information on every public parameter for each method – includes the parameter name and type.
§ Information on public enumerations including names and values.
§ Information on the assembly version (each assembly has a specific version number).
§ Intermediate language code to execute.
§ Required resources such as pictures, assembly metadata – also called the assembly manifest (the assembly title, description, version information, etc).
Multiple versions of an assembly can run simultaneously on the same client computer. This aids with compatibility with previous versions.
Assemblies shared by multiple applications on a client computer can be installed into the global assembly cache of Windows – this enhances security because only users with Administrator privileges on the machine can delete from the global assembly cache.
Strong-Named Assemblies
The strong-named assembly concept is used to guarantee the uniqueness of an assembly name – unique names are generated with the use of public and private key pairs when an assembly is compiled.
Applications can generally only run with the assembly version with which they are originally compiled. In order to update a component (such as a DLL for a control you've created), a publisher policy file is used to redirect an assembly binding request to a new version.
The .NET framework checks the integrity of strong-named assemblies to ensure they have not been modified since they were last built. This prevents unauthorized modification before loading.
The .NET framework creates a strong-named assembly by combining the assembly identify (name, version, and culture information) with a public key and digital signature.
As the programmer, you must generate the strong name key file (.snk filename extension) that contains the public-private key pair by using the Strong Name (Sn.Exe) utility or Visual Basic.NET IDE (this latter choice is usually the approach taken – simply involves clicking the right block during the building of an assembly). In fact, a project's property page has a Strong Name section to automatically generate a strong name key file to add to a project. The public key is inserted into an assembly at compile time. The private key is used to sign the assembly.
Versioning Strong-Named Assemblies
This shows an example publisher policy file written in XML. This file would be compiled for shipment with a new component version using the Assembly Generation tool (Al.Exe). This signs the assembly with the strong name used originally with the first version in order to confirm that the new component is from a valid source.
(configuration)
(runtime)
(assemblyBinding)
(dependentAssembly)
(assemblyIdentity name = "myassembly"
publicKeyTokey="ba76ce45677f9cd6"
culture="en-us"/)
(bindingRedirect
oldVersion="1.0.0.0"
newVersion="2.0.0.0"/)
(codeBase version="2.0.0.0"
href=http://www.fh.ora2003.edu/Test.dll/)
(/dependentAssembly)
(/assemblyBinding)
(/runtime)
(/configuration)
Ooops :-( i was unable to write less than sign and greater than sign in this blog so i had to use
( - less than sign
) - greater than sign.
The publicKeyTokey attribute is a hexadecimal value that identifies the strong name of the assembly.
Tuesday, February 28, 2006
How to send e-mail programmatically with System.Web.Mail and Visual Basic .NET
This article demonstrates how to use System.Web.Mail to send an e-mail message in Visual Basic .NET.
1.Start Microsoft Visual Studio .NET. On the File menu, click New and then click Project. Click Visual Basic Projects, click the Console Application template, and then click OK. By default, Module1.vb is created.
2.Add a reference to System.Web.dll. To do this, follow these steps:
a.On the Project menu, click Add Reference.
b.On the .NET tab, locate System.Web.dll, and then click Select.
c.Click OK in the Add References dialog box to accept your selections. If you receive a prompt to generate wrappers for the libraries you selected, click Yes.
3.In the code window, replace the whole code with:Imports System.Web.Mail
Module Module1
Sub Main()
Dim oMsg As MailMessage = New MailMessage()
' TODO: Replace with sender e-mail address.
oMsg.From = "sender@somewhere.com"
' TODO: Replace with recipient e-mail address.
oMsg.To = "recipient@somewhere.com"
oMsg.Subject = "Send using Web Mail"
' SEND IN HTML FORMAT (comment this line to send plain text).
oMsg.BodyFormat = MailFormat.Html
'HTML Body (remove HTML tags for plain text).
oMsg.Body = "'Hello World!'" ' you can use html tags here to edit your texts as you wish
' ADD AN ATTACHMENT.
' TODO: Replace with path to attachment.
Dim sFile As String = "C:\temp\Hello.txt"
Dim oAttch As MailAttachment = New MailAttachment(sFile, MailEncoding.Base64)
oMsg.Attachments.Add(oAttch)
' TODO: Replace with the name of your remote SMTP server.
SmtpMail.SmtpServer = "MySMTPServer"
SmtpMail.Send(oMsg)
oMsg = Nothing
oAttch = Nothing
End Sub
End Module
4.Modify code where you see "TODO".
5.Press F5 to build and run the program.
6.Verify that the e-mail message has been sent and received.
1.Start Microsoft Visual Studio .NET. On the File menu, click New and then click Project. Click Visual Basic Projects, click the Console Application template, and then click OK. By default, Module1.vb is created.
2.Add a reference to System.Web.dll. To do this, follow these steps:
a.On the Project menu, click Add Reference.
b.On the .NET tab, locate System.Web.dll, and then click Select.
c.Click OK in the Add References dialog box to accept your selections. If you receive a prompt to generate wrappers for the libraries you selected, click Yes.
3.In the code window, replace the whole code with:Imports System.Web.Mail
Module Module1
Sub Main()
Dim oMsg As MailMessage = New MailMessage()
' TODO: Replace with sender e-mail address.
oMsg.From = "sender@somewhere.com"
' TODO: Replace with recipient e-mail address.
oMsg.To = "recipient@somewhere.com"
oMsg.Subject = "Send using Web Mail"
' SEND IN HTML FORMAT (comment this line to send plain text).
oMsg.BodyFormat = MailFormat.Html
'HTML Body (remove HTML tags for plain text).
oMsg.Body = "'Hello World!'" ' you can use html tags here to edit your texts as you wish
' ADD AN ATTACHMENT.
' TODO: Replace with path to attachment.
Dim sFile As String = "C:\temp\Hello.txt"
Dim oAttch As MailAttachment = New MailAttachment(sFile, MailEncoding.Base64)
oMsg.Attachments.Add(oAttch)
' TODO: Replace with the name of your remote SMTP server.
SmtpMail.SmtpServer = "MySMTPServer"
SmtpMail.Send(oMsg)
oMsg = Nothing
oAttch = Nothing
End Sub
End Module
4.Modify code where you see "TODO".
5.Press F5 to build and run the program.
6.Verify that the e-mail message has been sent and received.
How To Create Classes and Objects in Visual Basic .NET
In Visual Basic .NET, a class can contain fields, methods, and properties. This article demonstrates how to create a new class to represent a baseball team. In this article, you will define fields, methods, and properties for the class. You will then create an object of this class type and make use of its methods and properties.
http://support.microsoft.com/kb/307210/en-us
http://support.microsoft.com/kb/307210/en-us
How to send attachments in an e-mail message by using Visual Basic .NET
To send attachments in an e-mail message by using Visual Basic .NET, follow these steps:
1.Start Microsoft Visual Studio .NET.
2.On the File menu, point to New, and then click Project.
3.In the Visual Basic Projects types list, click Console Application. By default, the Module1.vb file is created.
4.If Office Outlook 2003 is installed on your development computer, add a reference to the Microsoft Outlook 11.0 Object Library. If Outlook 2002 is installed on your development computer, add a reference to the Microsoft Outlook 10.0 Object Library. To do so, follow these steps:
a.On the Project menu, click Add Reference.
b.Click the COM tab, locate Microsoft Outlook 11.0 Library or Microsoft Outlook 10.0 Object Library, and then click Select.
c.In the Add References dialog box, click OK.
d.If you are prompted to generate wrappers for the libraries that you selected, click Yes.
5.In the code window, replace the code with the following:Module Module1
Sub Main()
' Create an Outlook application.
Dim oApp As Outlook._Application
oApp = New Outlook.Application()
' Create a new MailItem.
Dim oMsg As Outlook._MailItem
oMsg = oApp.CreateItem(Outlook.OlItemType.olMailItem)
oMsg.Subject = "Send Attachment Using OOM in Visual Basic .NET"
oMsg.Body = "Hello World" & vbCr & vbCr
' TODO: Replace with a valid e-mail address.
oMsg.To = "user@example.com"
' Add an attachment
' TODO: Replace with a valid attachment path.
Dim sSource As String = "C:\Temp\Hello.txt"
' TODO: Replace with attachment name
Dim sDisplayName As String = "Hello.txt"
Dim sBodyLen As String = oMsg.Body.Length
Dim oAttachs As Outlook.Attachments = oMsg.Attachments
Dim oAttach As Outlook.Attachment
oAttach = oAttachs.Add(sSource, , sBodyLen + 1, sDisplayName)
' Send
oMsg.Send()
' Clean up
oApp = Nothing
oMsg = Nothing
oAttach = Nothing
oAttachs = Nothing
End Sub
End Module
6.Search for the TODO text string in the code, and then modify the code for your environment.
7.Press the F5 key to build and to run the program.
8.Make sure that the e-mail message and the attachment have been sent.
1.Start Microsoft Visual Studio .NET.
2.On the File menu, point to New, and then click Project.
3.In the Visual Basic Projects types list, click Console Application. By default, the Module1.vb file is created.
4.If Office Outlook 2003 is installed on your development computer, add a reference to the Microsoft Outlook 11.0 Object Library. If Outlook 2002 is installed on your development computer, add a reference to the Microsoft Outlook 10.0 Object Library. To do so, follow these steps:
a.On the Project menu, click Add Reference.
b.Click the COM tab, locate Microsoft Outlook 11.0 Library or Microsoft Outlook 10.0 Object Library, and then click Select.
c.In the Add References dialog box, click OK.
d.If you are prompted to generate wrappers for the libraries that you selected, click Yes.
5.In the code window, replace the code with the following:Module Module1
Sub Main()
' Create an Outlook application.
Dim oApp As Outlook._Application
oApp = New Outlook.Application()
' Create a new MailItem.
Dim oMsg As Outlook._MailItem
oMsg = oApp.CreateItem(Outlook.OlItemType.olMailItem)
oMsg.Subject = "Send Attachment Using OOM in Visual Basic .NET"
oMsg.Body = "Hello World" & vbCr & vbCr
' TODO: Replace with a valid e-mail address.
oMsg.To = "user@example.com"
' Add an attachment
' TODO: Replace with a valid attachment path.
Dim sSource As String = "C:\Temp\Hello.txt"
' TODO: Replace with attachment name
Dim sDisplayName As String = "Hello.txt"
Dim sBodyLen As String = oMsg.Body.Length
Dim oAttachs As Outlook.Attachments = oMsg.Attachments
Dim oAttach As Outlook.Attachment
oAttach = oAttachs.Add(sSource, , sBodyLen + 1, sDisplayName)
' Send
oMsg.Send()
' Clean up
oApp = Nothing
oMsg = Nothing
oAttach = Nothing
oAttachs = Nothing
End Sub
End Module
6.Search for the TODO text string in the code, and then modify the code for your environment.
7.Press the F5 key to build and to run the program.
8.Make sure that the e-mail message and the attachment have been sent.
Wednesday, February 22, 2006
Life is a....
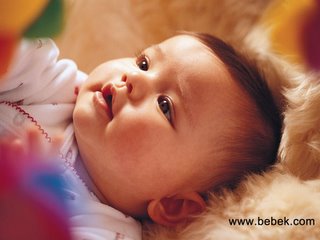
Life is a challenge
Meet it
Life is a gift
Accept it
Life is an adventure
Dare it
Life is a sorrow
Face it
Life is a duty
Perform it
Life is a mystery
Unfold it
Life is a game
Play it
Life is a song
Sing it
Life is an opportunity
Take it
Life is a journey
Complete it
Life is a promise
Fulfill it
Life is a love
Love it
Life is a beauty
Praise it
Life is a spirit
Realize it
Life is a struggle
Fight it
Life is a puzzle
Solve it
Life is a goal
Achieve it
Subscribe to:
Posts (Atom)